Android生命周期
onCreate–创建Activity时调用
onStart–当Activity界面变为用户可见时调用
onResume–当Activity界面获取到焦点时调用
onPause–当Activity失去焦点时调用
onStop–当Activity变为不可见时调用
onDestory–当Activity被销毁时调用
onRestart–当Activity再次启动时调用
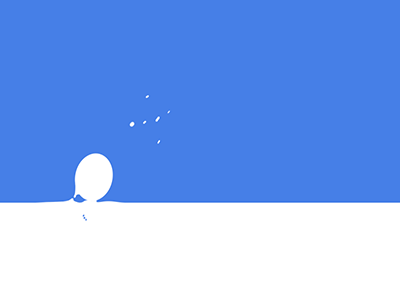
启动模式概念
决定生成新的Activity实例是否重用已存在的Activity实例,是否与其他Activity实例共用一个Task。
Task概念
Task是一个栈结构的对象,一个task可以管理多个Activity,启动一个应用,也就创建一个相应的Task。
Task是一个具有栈结构的容器,可以放置多个Activity实例。
启动一个应用,系统就会为之创建一个Task,来存放根Activity。
一个Activity启动另一个Activity时,两者是存放在同一个Task中的,后者被压入前者的task栈,用户按下回退键,后者从task被弹出,前者又显示出来。
Android:taskAffinity
指出了他希望进入的task。如果一个Activity没有显式的指明Activity的taskAffinity,那么它的这个属性就等于Application指明的taskAffinity。如果Application也没有指明,那么该taskAffinity的值就等同于包名。Task也有自己的Activity属性,它的值就等于它的根Activity的taskAffinity的值。
四种启动模式
standard
默认的启动模式,每次激活Activity(startActivity)时,都创建Activity实例,并放入任务栈中。
singleTop
如果激活一个Activity,首先判断此Activity是否在栈顶。若是则不需要创建实例,其他情况都需要创建。
singleTask
激活一个Activity,判断任务栈中是否存在该实例,若有,则不需要创建,只需要放入栈顶,并把此Activity之上的实例都出栈。
singleInstance
只有一个实例,并且这个实例独立运行在一个Task中,这个Task只有这个实例,不允许有其他Activity存在。
IntentFlag的常用值
FLAG_ACTIVITY_NEW_TASK
系统会寻找或创建一个新的task来放置目标Activity,查找时依据目标Activity的taskAffinity属性进行匹配,如果找到一个task的taskAffinity与之相同,就将目标压入此task中。若没有找到,则创建一个新的task,并将task的taskAffinity设置为目标Activity的taskAffinity,将目标Acctivity放入此task中。
FLAG_ACTIVITY_SINGLE_TOP
当task中存在目标Activity实例并且位于栈的顶端时,不再创建新的,直接利用这个实例。
FLAG_ACTIVITY_CLEAR_TOP
如果要激活的那个Activity在任务栈中存在实例,则不需要创建,只需要把实例放入栈顶,并把它之上的实例都出栈。
FLAG_ACTIVITY_REORDER_TO_FRONT
如果栈已经存在,则将它拿到栈顶,不会启动新Activity。
intent传参方式与Bundle传参方式
AndroidManifest.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.inter">
<application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.MApplication"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> //添加布局文件 <activity android:name=".InterActivity"> </activity> </application>
</manifest>
|
MainActivity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| package com.example.inter;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button;
public class MainActivity extends AppCompatActivity {
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button button = (Button) findViewById(R.id.btn1); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent = new Intent(MainActivity.this,InterActivity.class);
Bundle bundle = new Bundle(); bundle.putString("name","abcde"); bundle.putInt("age",18); intent.putExtras(bundle); startActivity(intent); } }); } }
|
InterActivity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| package com.example.inter;
import android.app.Activity;
import android.content.Intent; import android.os.Bundle;
import android.widget.TextView;
import androidx.annotation.Nullable;
public class InterActivity extends Activity { @Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.inter_layout); TextView textView = (TextView) findViewById(R.id.text1); TextView int1 = (TextView) findViewById(R.id.int1);
Intent intent = getIntent(); Bundle bundle = intent.getExtras(); String name = bundle.getString("name"); int age = bundle.getInt("age"); textView.setText(name); int1.setText(age+""); } }
|
activity_main.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity">
<TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> <Button android:id="@+id/btn1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="带参数传递" />
</androidx.constraintlayout.widget.ConstraintLayout>
|
inter_layout.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/text1" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:id="@+id/int1" android:layout_width="wrap_content" android:layout_height="wrap_content" />
</LinearLayout>
|